Exploring Innovative Uses of Lists in Python Programming
Written on
Understanding Lists in Python
In Python, a list is a versatile data structure that consists of an ordered collection of elements. Lists are mutable, meaning you can modify their contents, and they can hold elements of differing data types, including other lists.
Creating a List
Lists can be created in two primary ways: by using square brackets [] and placing elements separated by commas, or through the built-in function list(iterable).
If you're just starting with built-in functions and iterables in Python, you might find these resources helpful:
- Namespaces & Scopes: An Easy Lesson In Python - Essential knowledge for Python developers!
levelup.gitconnected.com
- But How Do Loops Work In Python? - Dive deep into the world of iteration!
levelup.gitconnected.com
Let’s explore some advanced techniques for working with lists in Python!
Creating Lists with List Comprehensions
List comprehensions offer a swift and concise method for generating lists. For example:
list_of_squares = [i**2 for i in range(10)]
This approach is generally faster than using a traditional for loop with appending elements to an empty list. Let’s observe this in practice!
Nested Lists: A Powerful Feature
Lists can also contain other lists, enabling you to represent complex data structures efficiently. For instance, we can create a 3x3 matrix using nested lists.
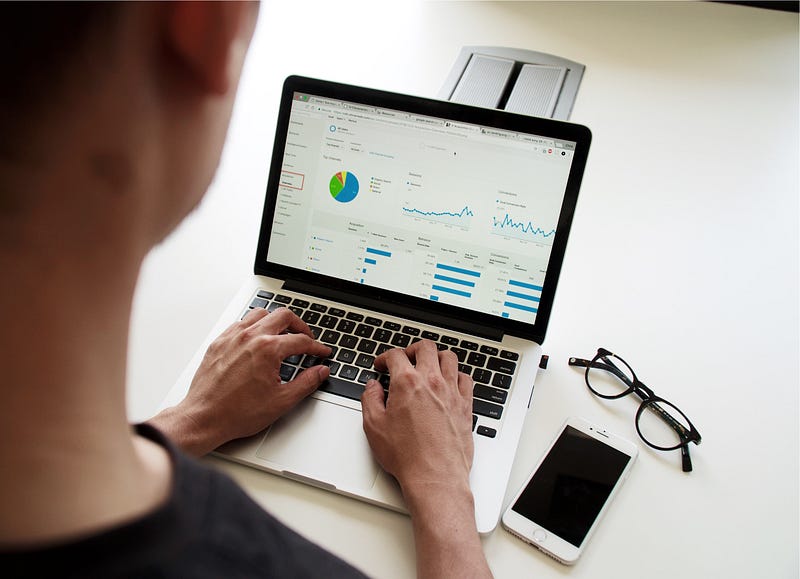
Lists as Stacks
A stack is a data structure that follows a LIFO (last in, first out) methodology. Imagine a stack of books; you can only add or remove the top book. This means the last book added will be the first one to be taken out.
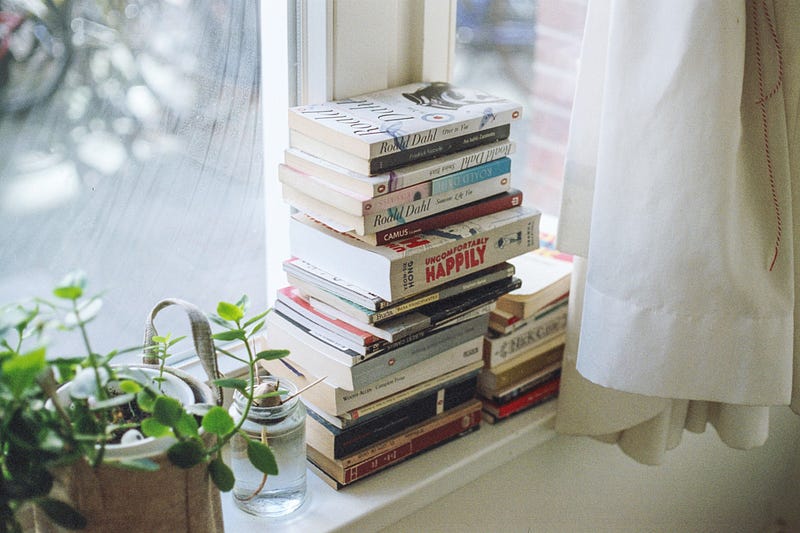
We can implement this behavior in Python using the append and pop methods.
Lists as Queues
Conversely, a queue is structured to allow FIFO (first in, first out) access. Picture a line of shoppers at a store; the first person to enter the queue is the first to leave.
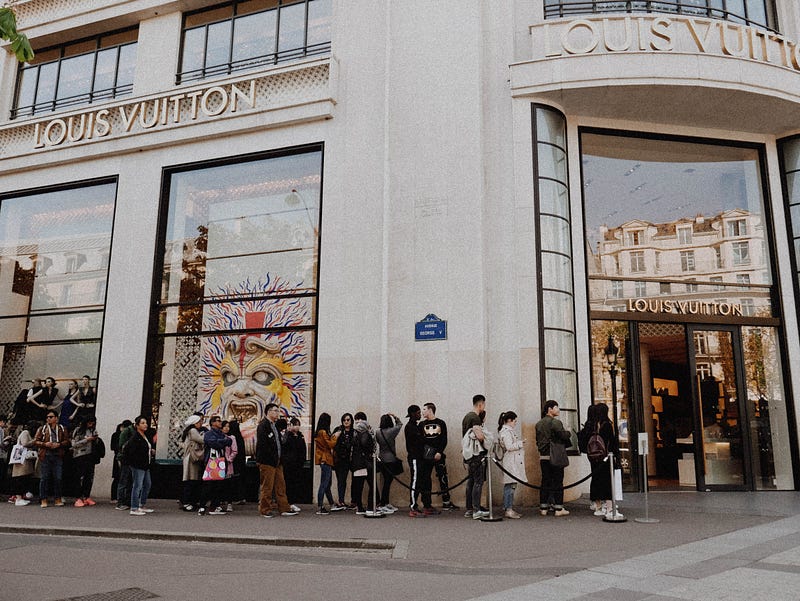
Challenges with Using Lists as Queues
While adding and removing elements from the end of a list is efficient, performing these actions from the beginning can slow down the process significantly, as all subsequent elements must shift in memory.
Solution
To address this limitation, Python offers a specialized collection known as deque. You can import this from the built-in collections module for an optimized list-like structure.
We hope this guide helps you discover new aspects of Python lists! Thank you for taking the time to read this article!
Explore more insights from Dr. Ashish Bamania and many others on Medium. Your membership contributes to the community's growth.
Level Up Coding
Thanks for being part of our community! Before you leave:
- Clap for this story and follow the author
- Check out more content in the Level Up Coding publication
- Join the Level Up talent collective to find amazing job opportunities!