Getting Started with Python: A Comprehensive Beginner's Overview
Written on
Chapter 1: Introduction to Python
Python stands out as a versatile, high-level programming language extensively utilized in web development, data analysis, artificial intelligence, and scientific computing. Its straightforward syntax, extensive libraries, and robust community support make it an excellent choice for novices. This article will delve into essential concepts and features of Python that will set you on the path to programming.
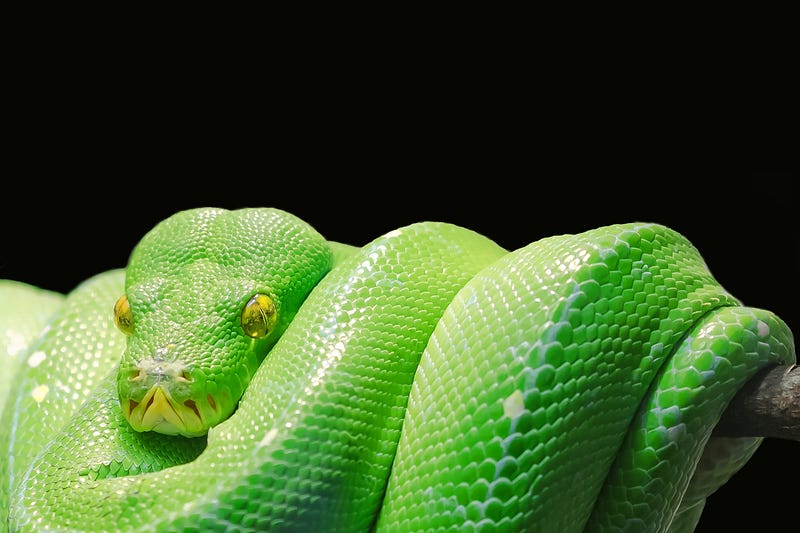
First, let's explore Python's fundamental syntax. Unlike many programming languages that use curly braces or keywords like "begin" and "end," Python employs indentation to define code blocks. This makes the amount of whitespace at the start of a line crucial, indicating the nesting level within a block of code. For instance, consider the following function that takes two inputs and returns their sum:
def add(a, b):
return a + b
In this example, the code block inside the function is indented by four spaces, a common practice in Python. However, any consistent spacing can be utilized within the same block.
Next, we will examine data types. Python includes several built-in types such as integers, floats, strings, and booleans. An integer represents a whole number, a float has a decimal point, a string consists of a sequence of characters, and a boolean can be either True or False. Here are some examples:
x = 3 # integer
y = 3.14 # float
z = "hello" # string
b = True # boolean
Python also offers various built-in data structures, including lists, tuples, and dictionaries. A list is an ordered collection, a tuple is an immutable version of a list, and a dictionary consists of key-value pairs. Below are examples of each structure:
# list
lst = [1, 2, 3, 4, 5]
# tuple
tpl = (1, 2, 3, 4, 5)
# dictionary
dct = {'a': 1, 'b': 2, 'c': 3}
You can access elements in a list or tuple through indexing and retrieve dictionary elements using their keys.
Along with these foundational data types and structures, Python provides numerous built-in functions and modules for various tasks. For instance, the print() function displays text on the screen, while len() determines the length of a string or list. The math module includes functions for mathematical operations, such as sqrt() and pow().
Python also supports control structures like if-else statements, for loops, and while loops, which help manage the program's flow more effectively. Here’s an example:
x = 5
if x > 3:
print("x is greater than 3")
elif x < 3:
print("x is less than 3")
else:
print("x is equal to 3")
for i in range(5):
print(i)
i = 0
while i < 5:
print(i)
i += 1
Additionally, Python boasts a wealth of libraries, including NumPy and Pandas, commonly used for data analysis and scientific tasks, as well as TensorFlow and PyTorch for machine learning and deep learning. These libraries offer an array of tools and functions to execute complex tasks with minimal code.
An essential aspect of Python is its support for object-oriented programming (OOP). OOP is a paradigm that models real-world entities and their interactions through classes and objects. Classes define an object's properties and methods, while objects are specific instances of these classes. Here’s a simple class example:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print("Woof!")
In this instance, we created a class named "Dog" with two attributes (name and breed) and one method (bark). To instantiate this class, you can use:
dog1 = Dog("Fido", "Golden Retriever")
In summary, Python is a robust and adaptable programming language that is straightforward to learn and apply. Its simple syntax, extensive libraries, and strong community support make it a fantastic option for beginners. We have discussed various essential concepts and features of Python to help you embark on your programming journey. Remember to practice regularly and feel free to experiment with different libraries and modules to enhance your Python skills.
More content at PlainEnglish.io. Sign up for our free weekly newsletter. Follow us on Twitter, LinkedIn, YouTube, and Discord.
Chapter 2: Video Resources
This video titled "Python for Beginners - Learn Python in 1 Hour" provides a quick yet comprehensive introduction to the Python programming language, ideal for those starting their coding journey.
The second video, "Python Tutorial for Absolute Beginners #1 - What Are Variables?", focuses on the essential concept of variables in Python, perfect for absolute beginners.