Effective String Concatenation Techniques in Python
Written on
Chapter 1: Introduction to String Concatenation
When I began my journey with Python, I frequently relied on the + operator to concatenate strings—a method many of us find straightforward and intuitive. However, it’s important to realize that this method is not considered Pythonic, and its performance pales in comparison to other approaches available.
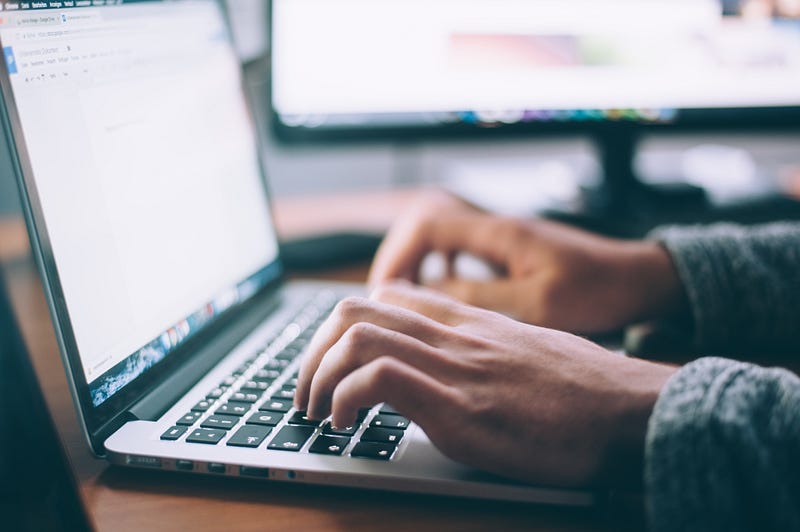
In this article, I will examine various techniques for joining strings and highlight their differences.
Section 1.1: The + Operator
Most people are familiar with this approach, as it is also common in various other programming languages. Here’s a simple example:
str_1 = 'Python'
str_2 = 'Programming Language'
print(str_1 + ' ' + str_2) # Output: Python Programming Language
Seems straightforward, right? But what happens when we have a list of strings? In such cases, a for-loop may be employed:
strs = ['Join', 'strings', 'in', 'Python']
result = ''
for s in strs:
result += ' ' + s
result = result[1:] # Removing the leading space
print(result) # Output: Join strings in Python
Section 1.2: The join() Method
The join() method offers a way to concatenate a sequence of strings using a specified delimiter. This delimiter is passed to the join() method as an argument:
strs = ['Join', 'strings', 'in', 'Python']
result = ' '.join(strs)
print(result) # Output: Join strings in Python
Section 1.3: The % Operator
The % operator is excellent for string formatting and can also be utilized for string concatenation:
platform = "Medium"
month = 10
print("I have been a writer on %s for %d months." % (platform, month))
# Output: I have been a writer on Medium for 10 months.
Here, %s is used for strings and %d for integers. The variables platform and month are provided as a tuple and inserted into the string at the corresponding placeholders. You can also combine % with join to concatenate a list of strings:
cities = ["Hanoi", "Munich", "Nuremberg"]
print("I have lived in %s." % ', '.join(cities))
# Output: I have lived in Hanoi, Munich, Nuremberg.
Section 1.4: str.format()
The str.format() method replaces placeholders in a string with corresponding values. Placeholders are denoted by curly braces {} and can be filled with either positional or keyword arguments:
platform = "Medium"
month = 10
print("I have been a writer on {} for {} months.".format(platform, month))
# Output: I have been a writer on Medium for 10 months.
print("I have been a writer on {p} for {m} months.".format(m=month, p=platform))
# Output: I have been a writer on Medium for 10 months.
Similar to the % operator, if concatenating a list of strings, join is employed:
cities = ["Hanoi", "Munich", "Nuremberg"]
print("I have lived in {}.".format(', '.join(cities)))
# Output: I have lived in Hanoi, Munich, Nuremberg.
Section 1.5: f-strings
Formatted string literals, or f-strings, allow you to embed expressions inside string literals. This feature is available in Python 3.6 and later:
platform = "Medium"
month = 10
print(f"I have been a writer on {platform} for {month} months.")
# Output: I have been a writer on Medium for 10 months.
You can also join a list of strings with f-strings:
cities = ["Hanoi", "Munich", "Nuremberg"]
print(f"I have lived in {', '.join(cities)}.")
# Output: I have lived in Hanoi, Munich, Nuremberg.
Chapter 2: Performance Comparison
In this section, I will use timeit to compare the efficiency of the + operator with join() when concatenating a list containing 1,000,000 elements:
strings = ['a'] * 1_000_000
def join_with_plus(strs: list) -> str:
result = ''
for s in strs:
result += sreturn result
def join_with_join(strs: list) -> str:
return ''.join(strs)
print(join_with_plus(strs=strings) == join_with_join(strs=strings))
# Output: True
%timeit join_with_plus(strs=strings)
# 94.3 ms ± 3.12 ms per loop (mean ± std. dev. of 7 runs, 10 loops each)
%timeit join_with_join(strs=strings)
# 9.1 ms ± 1.07 ms per loop (mean ± std. dev. of 7 runs, 100 loops each)
The results are quite clear: join() is significantly faster for large lists of strings. The + operator is slower because it creates a new string object each time it is utilized, which leads to the creation of numerous temporary string objects.
The join() method, on the other hand, operates by creating a single string buffer and appending each string to it, thus avoiding the overhead of creating multiple temporary objects.
When it comes to readability, a one-liner using join is definitely preferable over the for-loop approach with the + operator.
Chapter 3: Readability and Performance Evaluation
In terms of readability, f-strings outperform the other methods. The clarity of embedding expressions directly within the string makes it much easier to understand the relationships between the expressions and the resulting output. Str.format() takes the second place thanks to its placeholders, which reduce the likelihood of errors compared to the % operator.
From a performance standpoint, f-strings are superior to str.format(), while the % operator comes in last. The reason behind this is that f-strings evaluate expressions at runtime, avoiding the need for additional function calls or string concatenation, thus minimizing overhead and memory allocations.
In summary, the most efficient methods for string concatenation in Python are:
- join() is preferred over the +
- f-strings are superior to str.format() and the % operator
This video titled "Coffee Compiler Club, 2021_01_15" provides additional insights into string operations in Python.
Thank you for being part of our community! If you enjoyed this article, please consider giving a clap and following the author. Explore more content on Level Up Coding, and join us on Twitter, LinkedIn, or our Newsletter.